If you’ve seen the error “cl_utils.lua:46: bad argument“, you’re probably frustrated, confused, and wondering how to fix it.
This issue pops up more often than you’d think, especially for developers working with Lua scripts.
In this article, I’m going to explain what this error means, why it happens, and most importantly, how to fix it. I’ll keep the tech talk minimal, but enough so you get exactly what’s going on.
Let’s dive right in.
What Does “cl_utils.lua:46: bad argument” Mean?
This error is a classic Lua runtime error.
In plain terms, “cl_utils.lua:46: bad argument” is the Lua interpreter’s way of saying, “Hey, the function you’re trying to call isn’t getting the type of argument it expects.”
The “46” refers to the line in the cl_utils.lua file where the problem is happening.
But what causes this mismatch? More often than not, it’s a case of the function receiving data in the wrong format.
For example:
- You might be passing a string where the function needs a number.
- Or, you’re giving a nil value when the function expects something concrete like a table.
This is a simple problem, but it can be a headache when you’re in the middle of coding and this error just won’t leave you alone.
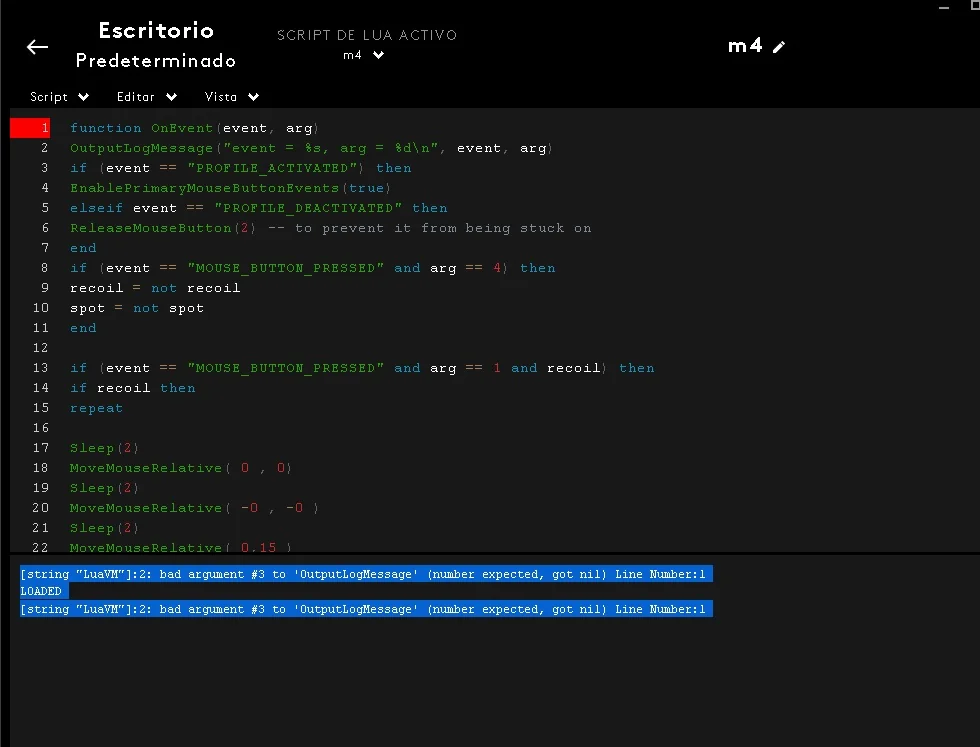
A Real-Life Example of cl_utils.lua:46: bad argument
Imagine you’re coding a game using Lua. You’ve written a function that calculates the score for a player based on their in-game actions.
You pass in the player’s name and expect to see their score show up.
Boom! Instead, you get “cl_utils.lua:46: bad argument” flashing across your screen.
What happened?
Your function was expecting a number (the player’s current score), but you passed it a string (the player’s name).
Lua doesn’t know what to do with that string, so it throws a fit and gives you the cl_utils.lua:46: bad argument error.
This type of thing happens all the time in Lua scripts. It’s easy to mix up your arguments when coding, especially in larger projects.
Common Causes of the “cl_utils.lua:46: bad argument” Error
Now that we know what the error means, let’s talk about what typically causes it.
- Mismatched Data Types
The most common reason for the “cl_utils.lua:46: bad argument” error is passing a variable of the wrong type to a function.
For example:- Passing a string when a number is expected.
- Passing a nil value instead of an actual object.
- Missing Required Arguments
Sometimes you’ll forget to pass an argument entirely, leaving a function hanging. If the function needs more than one argument, leaving one out will trigger this error. - Incorrect Order of Arguments
In Lua, the order in which you pass arguments matters. If you mix up the order, you might accidentally pass a string where a table is expected, or a number where a boolean is required. - Nil Values
Passing a nil value when the function expects something else is a sure-fire way to get this error. This happens when a variable hasn’t been initialized or you’ve accidentally wiped it clean.
How to Fix the “cl_utils.lua:46: bad argument” Error
Here’s the good part—fixing “cl_utils.lua:46: bad argument” is usually straightforward.
Follow these steps, and you should be back on track quickly:
1. Double-Check Your Arguments
Go back to the line that calls the function and carefully check what arguments you’re passing. Are they the right types?
- If the function needs a number, pass a number.
- If it needs a table, pass a table.
2. Add Print Statements for Debugging
Sometimes you need a little help figuring out where things went wrong. Add print() statements right before your function calls to see exactly what values are being passed.
For example:
3. Check for Nil Values
Run a quick check to make sure all your variables have been initialized properly.
For example:
4. Review the Function Definition
Sometimes the error comes from not fully understanding what the function is supposed to do.
- Open the function definition and make sure you’re clear on what arguments it needs.
- Check the expected data types for each argument.
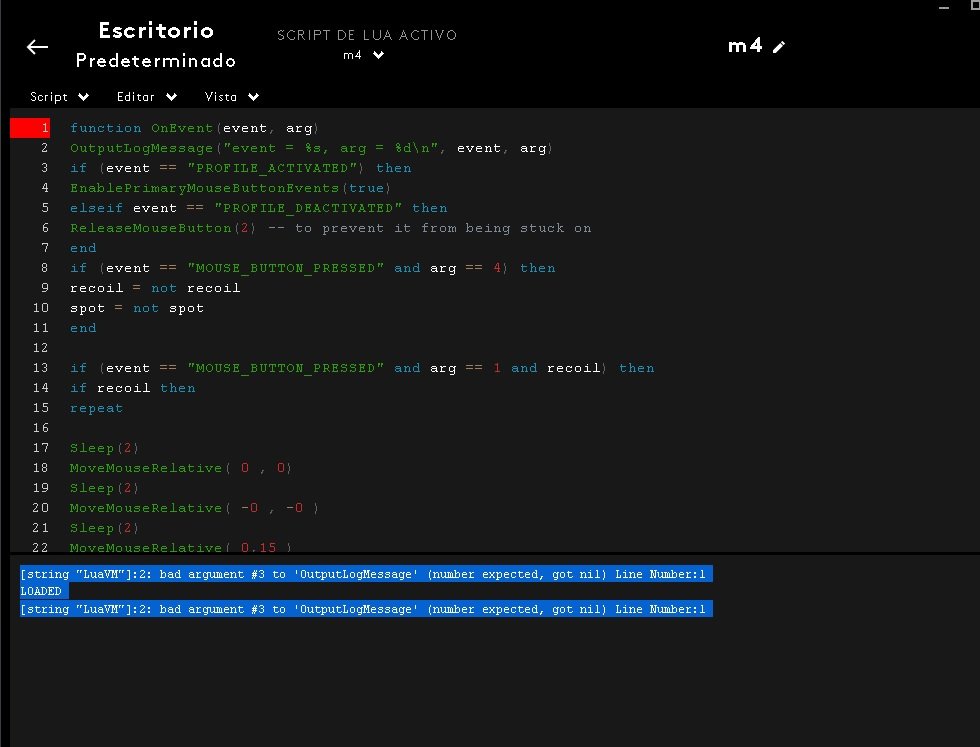
FAQs About cl_utils.lua:46: bad argument
Q: Why am I getting the cl_utils.lua:46: bad argument error out of nowhere?
A: This can happen when an uninitialized or nil value is accidentally passed to a function. It can also happen if a function’s parameters have changed, but you’re still passing the old arguments.
Q: Can I fix this error without changing the function?
A: Yes! If the function isn’t broken and it’s just the arguments you’re passing that are incorrect, you can fix the error by passing the correct values.
Q: Is this error specific to Lua, or can it happen in other programming languages?
A: While this exact error message is specific to Lua, the concept of passing wrong arguments or incorrect data types can happen in many programming languages, like Python, JavaScript, and C++.
Q: Does the “46” in the error message matter?
A: Yes! The “46” tells you the exact line in the cl_utils.lua file where the error occurred. It’s a helpful pointer for tracking down the issue.
Wrapping It Up
“cl_utils.lua:46: bad argument” might seem daunting at first, but it’s really just Lua’s way of pointing out an argument mismatch.
Whether it’s a data type problem, a nil value, or a simple argument mix-up, the solution usually lies in checking what you’re passing to the function.
Remember: Lua is picky about arguments, so always double-check that you’re passing exactly what the function expects.
So next time you see “cl_utils.lua:46: bad argument”, don’t panic. Just follow the steps in this guide, and you’ll have it sorted in no time.
Final Thought
At the end of the day, errors like “cl_utils.lua:46: bad argument” are just part of the learning process. They might slow you down, but they’ll ultimately help you write better, cleaner code.
And there you have it—next time you encounter “cl_utils.lua:46: bad argument”, you’ll know exactly what to do!